Unlock the Power of Google Docs with AI: A Step-by-Step Guide
Written on
Chapter 1: Enhancing Your Writing Experience
Integrating OpenAI's API into Google Docs is remarkably straightforward, enabling users to tap into the capabilities of GPT-3 for various writing tasks. With just a bit of coding in Google Apps Script, you can generate complete articles across multiple niches in mere minutes.
Do you think this sounds unrealistic? Thankfully, it's not. Recent innovations like ChatGPT have made large language models (LLMs) exceptionally powerful and accessible, often at no cost. By creating a new account with OpenAI, you can receive $18 in free credits, potentially allowing for the creation of thousands of articles using your AI-optimized text editor.
There’s no need to invest in costly AI writing tools or subscriptions when you can replicate their functionalities with just a few lines of custom code in Google Docs. As I mentioned, it's incredibly simple, and you won't need any advanced programming skills—just the ability to copy, paste, and read. I'll guide you through the entire process.
If you prefer not to read through the details, there's a complete code sample provided at the end of the article. However, if you're keen to stay updated on AI topics, follow me for insights that will help you become an AI expert.
Getting Your OpenAI API Key
OpenAI is the organization behind ChatGPT and its predecessor, GPT-3, which remains a powerful LLM. Although ChatGPT isn't yet available through the OpenAI API, I will update this guide once it becomes accessible.
Utilizing the OpenAI API allows us to leverage their advanced models in various applications. Rumor has it that GPT-4, an even more sophisticated model, will be released soon, but for the time being, we'll focus on enhancing Google Docs with GPT-3.
To begin, you'll need to create an OpenAI account and obtain your API key from the API menu.
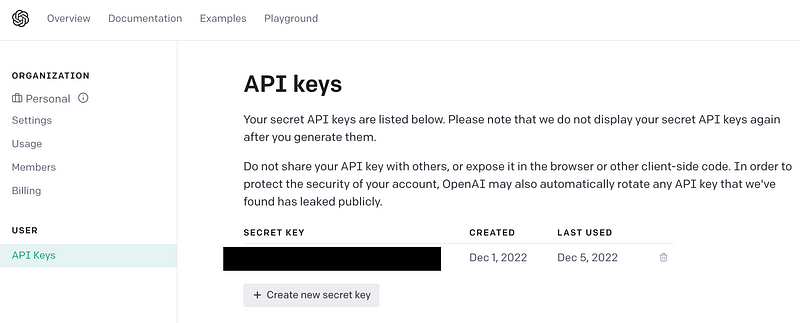
Where to Locate Your OpenAI Key
Once you have your API key, keep it handy as we will need it later. Now, let's turn our attention back to Google Docs.
Setting Up Google Docs for AI Integration
With your API key in hand, navigate to Google Docs and open the Apps Script editor.
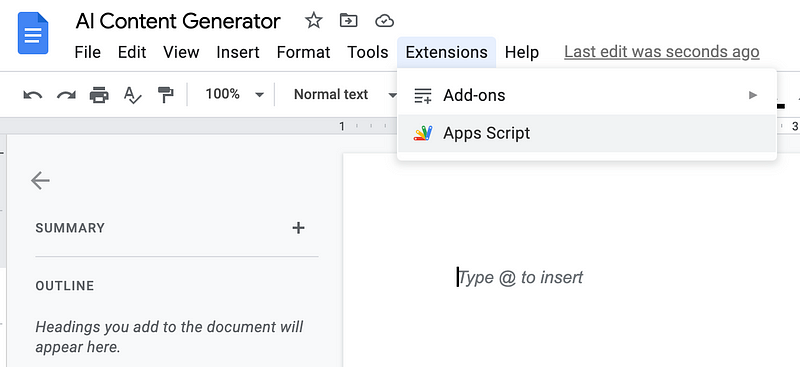
In the editor, you can remove the default code and input the following script:
function onOpen() {
DocumentApp.getUi().createMenu("GPT-3 Integration")
.addItem("Blog Ideas", "createBlogIdeas")
.addToUi();
}
This script creates a menu item for generating blog ideas. Save your changes and check the menu to see it in action.

Next, return to the Apps Script editor and add these two variables above the function:
var apiKey = "<Your OpenAI API Key>";
var model = "text-davinci-003";
The Davinci Model is OpenAI’s most advanced offering available through the API. You can learn more about other models and their pricing on their website.
At this point, we are ready to connect to the GPT-3 API.
Integrating Apps Script with OpenAI API
Now that we have our menu item and the foundational code, we can proceed to add functionality.
For instance, let's generate five blog ideas centered on utilizing AI within Google Docs. You can use any prompt, but here’s one to start: "Generate 5 blog ideas around how to integrate AI with Google Docs." Input this prompt into your Google Docs document.

Next, go back to the Apps Script and paste this code:
function createBlogIdeas() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
var prompt = selectedText;
temperature = 0;
maxTokens = 2060;
const requestBody = {
"model": model,
"prompt": prompt,
"temperature": temperature,
"max_tokens": maxTokens,
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
body.appendParagraph(json['choices'][0]['text']);
}
Now, wire this menu item to the function. Highlight the prompt you wrote in Google Docs and select your newly created menu item. After a brief wait, you should see something like this:

Impressive, right?
A Note: While I've kept this tutorial straightforward, GPT-3 is exceptionally powerful. Feel free to experiment in the playground to refine your prompts to suit your needs. Once you find a successful prompt, incorporate it and prepare for amazing results!
Further Enhancements with AI
Let’s add another function to our toolset. Back in Apps Script, highlight the menu, paste in a new menu item, and modify the command. Your menu code should now appear as follows:
function onOpen() {
DocumentApp.getUi().createMenu("GPT-3 Integration")
.addItem("Blog Ideas", "createBlogIdeas")
.addItem("Write Paragraph", "writeParagraph")
.addToUi();
}
To create a paragraph, highlight your text, select the new command, and copy and paste it. You can change the prompt to something like "Write a detailed paragraph about...".
Here’s the updated code:
function writeParagraph() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
var prompt = "Write a detailed paragraph about " + selectedText;
temperature = 0;
maxTokens = 2060;
const requestBody = {
"model": model,
"prompt": prompt,
"temperature": temperature,
"max_tokens": maxTokens,
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
body.appendParagraph(json['choices'][0]['text']);
}
Once completed, save your work and test it out.

Incredible, isn't it?
With this approach, you can craft various prompts for distinct purposes, such as writing conclusions, headlines, social media posts, SEO descriptions, and more. The key is to tailor your prompts according to your niche, as the quality of your output depends on the quality of your input. Experiment, iterate, and share your results—I would love to hear about your discoveries!
Bonus: Creating Images with DALL·E
If you wish to generate images, there's a slightly different approach, but it's just as easy! For images, you can choose sizes of 256x256, 512x512, or 1024x1024 pixels.
While I won't delve into it in this article, AI image generation has seen rapid advancements. Notable resources include DALL·E, Stable Diffusion, and MidJourney. I plan to explore this topic further in the future.
For now, let's stick with 512px images. Back in Apps Script, add the following to your menu:
function onOpen() {
DocumentApp.getUi().createMenu("GPT-3 Integration")
.addItem("Blog Ideas", "createBlogIdeas")
.addItem("Write Paragraph", "writeParagraph")
.addItem("Generate Image", "generateImage")
.addToUi();
}
For the function that generates images, use this code:
function generateImage() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
temperature = 0;
maxTokens = 2000;
var prompt = "Generate images for " + selectedText;
const requestBody = {
"prompt": prompt,
"n": 1,
"size": "512x512"
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
var url1 = json['data'][0]['url'];
body.appendImage(UrlFetchApp.fetch(url1).getBlob());
}
Notice that the OpenAI API endpoint differs, but you don’t need any additional settings as it generates images based on the selected text. Save it and let's run a test.

For instance, using the prompt “An open field with a single flower” could yield the following image:

When it comes to AI-generated images, the more detailed your prompt, the better the outcome. A reference guide from Merzmensch can help you optimize DALL·E image creation.
What Will You Create Using GPT-3 in Google Docs?
This concludes our introduction to utilizing GPT-3 within Google Docs at no cost. You may encounter some charges over time, but the initial $18 in free credits from OpenAI should be sufficient for your experimentation.
I hope you found this guide helpful and that the code doesn’t seem too daunting. If you experience any issues, don’t hesitate to leave a comment—I’m here to assist.
In future articles, I plan to delve into practical AI applications. I would love to hear about any creative ideas or interesting applications you want to see explored.
For those who just want the complete code, here it is:
var apiKey = "<Your Open AI API Key>";
var model = "text-davinci-003";
function onOpen() {
DocumentApp.getUi().createMenu("GPT-3 Integration")
.addItem("Blog Ideas", "createBlogIdeas")
.addItem("Write Paragraph", "writeParagraph")
.addItem("Generate Image", "generateImage")
.addToUi();
}
function createBlogIdeas() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
var prompt = selectedText;
temperature = 0;
maxTokens = 2060;
const requestBody = {
"model": model,
"prompt": prompt,
"temperature": temperature,
"max_tokens": maxTokens,
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
body.appendParagraph(json['choices'][0]['text']);
}
function writeParagraph() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
var prompt = "Write a detailed paragraph about " + selectedText;
temperature = 0;
maxTokens = 2060;
const requestBody = {
"model": model,
"prompt": prompt,
"temperature": temperature,
"max_tokens": maxTokens,
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
body.appendParagraph(json['choices'][0]['text']);
}
function generateImage() {
var doc = DocumentApp.getActiveDocument();
var selectedText = doc.getSelection().getRangeElements()[0].getElement().asText().getText();
var body = doc.getBody();
temperature = 0;
maxTokens = 2000;
var prompt = "Generate images for " + selectedText;
const requestBody = {
"prompt": prompt,
"n": 1,
"size": "512x512"
};
const requestOptions = {
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer " + apiKey
},
"payload": JSON.stringify(requestBody)
};
var responseText = response.getContentText();
var json = JSON.parse(responseText);
var url1 = json['data'][0]['url'];
body.appendImage(UrlFetchApp.fetch(url1).getBlob());
}
Thank you for reading!
For more content, visit PlainEnglish.io.
Subscribe to our free weekly newsletter and follow us on Twitter, LinkedIn, YouTube, and Discord.
Boost awareness and adoption for your tech startup with Circuit.
Explore how to supercharge your productivity with Typed, an advanced tool for Google Docs.
Learn how to seamlessly integrate ChatGPT with Google Docs for enhanced writing capabilities.