Mastering Vertical String Alignment in Python
Written on
Chapter 1: Introduction to String Alignment
In this guide, we will explore how to align strings vertically based on specific letters. The function align(strings, letter) takes a list of strings and a target letter, printing the strings so that the specified letters are aligned vertically. If a string does not contain the letter, it will be omitted from the output.
Here's an illustration of how the function operates:
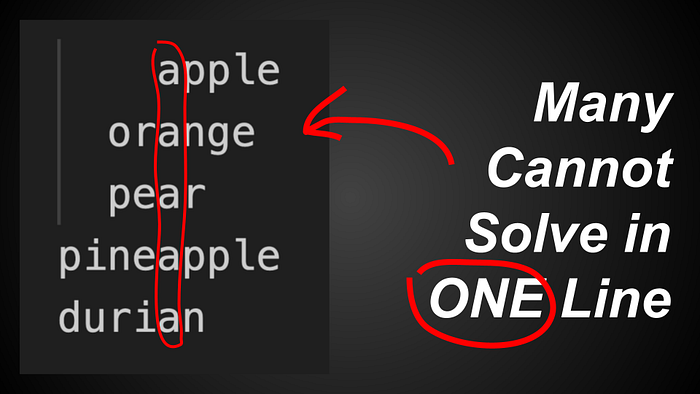
Let’s see an example with the letter 'a':
align(['apple', 'orange', 'pear', 'pineapple', 'durian'], 'a')
The output will be:
apple
orange
pear
pineapple
durian
Notice how the 'a's in each word are aligned by adding appropriate spaces in front of the strings.
Now, if we try with the letter 'e':
align(['apple', 'orange', 'pear', 'pineapple', 'durian'], 'e')
The result will be:
apple
orange
pearpineapple
Here, 'durian' is excluded because it does not contain 'e'.
Challenge: Create a One-Line Function
Before condensing our solution, let’s first write it out in a multi-line format.
Section 1.1: Initial Multi-Line Implementation
def align(strings, letter):
# Collect all indexes of the letter
indexes = []
for string in strings:
indexes.append(string.find(letter))
# Determine the maximum index of the letter
max_index = max(indexes)
# Print strings with the necessary spaces
for string in strings:
if letter in string:
num_spaces = max_index - string.find(letter)
print(num_spaces * ' ' + string)
This is our multi-line solution. Next, we will work on condensing it into a single line.
Section 1.2: Condensing to One Line
We can combine finding the maximum index and printing the strings into a single expression:
def align(strings, letter):
max_index = max([string.find(letter) for string in strings])
[print((max_index - string.find(letter)) * ' ' + string) for string in strings if letter in string]
To make the variable names shorter and the code cleaner, we can rename them:
def align(S, l):
M = max([s.find(l) for s in S])
[print((M - s.find(l)) * ' ' + s) for s in S if l in s]
Finally, we can force everything into one line using semicolons:
def align(S, l): M = max([s.find(l) for s in S]); [print((M - s.find(l)) * ' ' + s) for s in S if l in s]
And there you have it—a concise one-liner function for aligning strings!
Chapter 2: Video Examples and Further Learning
To further enhance your understanding, here are some helpful video resources:
The first video titled Aligning Strings Vertically (Can You Solve This in ONE Line? 4) covers the topic in detail and provides additional insights.
The second video, Aligning Inline Equations Vertically in Canvas, explores a related concept that may also be beneficial.
Conclusion
I hope this explanation has been clear and informative. If you found this content useful, consider showing your support by:
- Clapping for this story
- Leaving a comment with your thoughts
- Highlighting sections that resonated with you
These actions are greatly appreciated!