Understanding the Differences Between C++ and Python
Written on
Introduction to C++ and Python
Software development encompasses a variety of programming languages, among which C++ and Python are prominent.
C++, a middle-level programming language created by Bjarne Stroustrup, combines the capabilities of both low-level (such as drivers and kernel work) and high-level applications (like GUI and desktop software). By building upon the C language with object-oriented principles, C++ is often hailed as both powerful and user-friendly. Its popularity is evident, especially among software engineers, data analysts, and other professionals who appreciate its accessibility.
Conversely, Python is a versatile language widely used across different fields for tasks such as data analysis, automation, web development, and more. Thanks to its straightforward syntax, Python makes programming tasks more manageable, allowing users to focus on solving problems rather than wrestling with complex code.
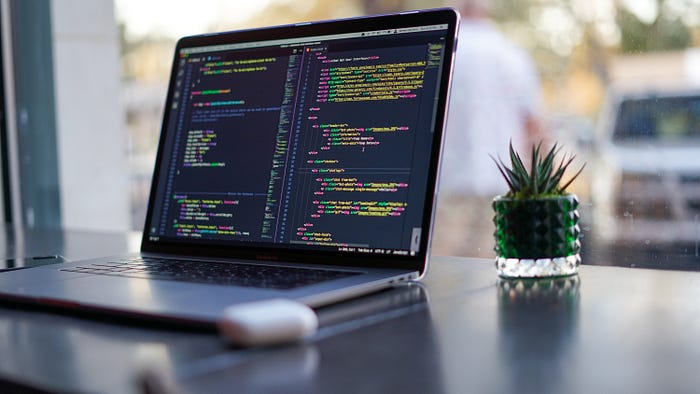
Key Differences Between C++ and Python
While both languages can achieve similar outcomes, they have notable differences worth considering:
1. Ease of Learning
Python's syntax resembles English, enhanced by indentation rules that simplify learning for beginners. In contrast, C++'s complex syntax—with its use of curly braces and semicolons—can be more challenging, making it less readable.
2. Compilation vs. Interpretation
Python is an interpreted language, requiring a runtime interpreter to execute code. In contrast, C++ is pre-compiled, allowing for quicker execution since it processes code through a compiler before runtime.
3. Speed
Due to its interpreted nature, Python is generally slower, as it determines data types during execution. C++, being pre-compiled, executes code more swiftly, benefiting from its robust object-oriented architecture.
4. Memory Management
Python offers dynamic memory management, automatically allocating and deallocating memory as needed. C++, however, requires manual memory management, which can lead to potential memory leaks if not handled correctly.
5. Variable Declaration
In Python, variables do not need explicit declaration before use, thanks to its dynamic typing. Conversely, C++ requires a variable's name and type to be declared, as it is statically typed.
6. Variable Scope
The scope of variables defines their accessibility within the code. While global and local scopes function similarly in both languages, Python allows variables to be accessed outside loops, whereas C++ restricts them within their respective loops.
7. Garbage Collection
Python includes built-in garbage collection that automatically frees memory when data is no longer in use. C++ lacks this feature, requiring manual memory deallocation.
8. Portability
Python is inherently portable, enabling code to run across different platforms without modification. C++, on the other hand, necessitates recompilation for different environments.
9. Rapid Prototyping
Python excels in rapid prototyping, allowing developers to quickly create functioning applications. C++ may prove to be more complex for such tasks due to its methodology.
10. Data Security
Python's default public access to class members and methods makes it less secure compared to C++, which employs strong data encapsulation through private access by default.
To illustrate the differences further, consider the bubble sort implementation in both languages:
Implementation of Bubble Sort in C++
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(arr[j], arr[j+1]);
}
Implementation of Bubble Sort in Python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
Conclusion
In summary, Python is ideal for rapid development and effective memory management, while C++ is preferred for speed and control over memory operations.
For more insights, connect with me on LinkedIn and Twitter.
Recommended Articles
- 8 Active Learning Insights of Python Collection Module
- NumPy: Linear Algebra on Images
- Exception Handling Concepts in Python
- Pandas: Dealing with Categorical Data
- Hyper-parameters: RandomSearchCV and GridSearchCV in Machine Learning
- Fully Explained Linear Regression with Python
- Fully Explained Logistic Regression with Python
- Data Distribution using Numpy with Python
- Decision Trees vs. Random Forests in Machine Learning
- Standardization in Data Preprocessing with Python
The first video titled "C vs Python: Which Is Better To Learn First?" explores the fundamental differences between C and Python, helping beginners decide which language to start with.
The second video, "From C to Python by Ross Rheingans-Yoo," provides insights on transitioning from C programming to Python, emphasizing the advantages of both languages.